10. Open Science#
Introduction
As medical research and collaborative platforms evolve, the Fena platform is pioneering the fusion of open science principles and secure Git practices. This dual commitment not only accelerates the sharing of scientific knowledge but also ensures that sensitive data remain shielded. At the heart of this balance is the belief that transparency doesn’t have to come at the expense of privacy.
Show code cell source
import networkx as nx
import matplotlib.pyplot as plt
# Set seed for layout
seed = 1234567890
# Directory structure
structure = {
"Challenges": ["Fraud", "Sloppiness", "Rigor", "Learning", "Truth", "Error"],
"Literacy": ["Grants", "Self-publish", "Knowledge-Gaps", "Open-Science", "Journals"],
"Numeracy": ["Stata", "Estimates", "R", "AI", "Python"],
"Project": ["Manuscripts", "Git", "Code"],
"Skills": ["Literacy", "Project", "Numeracy", "Workflow", "Challenges"],
"Workflow": ["High School Students", "Undergraduates", "Graduate Students", "Medical Students", "Residents", "Fellows", "Faculty", "Analysts", "Staff", "Collaborators", "Graduates"],
}
# Gentle colors for children
child_colors = ["lightgreen", "lightpink", "lightyellow", 'white', 'honeydew', 'lightcoral','azure', 'lightblue','lavender']
# List of nodes to color light blue
light_blue_nodes = ["Literacy", "Numeracy", "You", "Project", "Challenges"]
G = nx.Graph()
node_colors = {}
# Function to capitalize the first letter of each word
def capitalize_name(name):
return ' '.join(word.capitalize() for word in name.split(" "))
# Assign colors to nodes
for i, (parent, children) in enumerate(structure.items()):
parent_name = capitalize_name(parent.replace("_", " "))
G.add_node(parent_name)
# Set the color for Skills
if parent_name == "Skills":
node_colors[parent_name] = 'lightgray'
else:
node_colors[parent_name] = child_colors[i % len(child_colors)]
for child in children:
child_name = capitalize_name(child.replace("_", " "))
# Exclude "Co-Pilot" and "ChatGPT"
if child_name not in ["Co-Pilot", "ChatGPT"]:
G.add_edge(parent_name, child_name)
if child_name in light_blue_nodes:
node_colors[child_name] = 'lightblue'
else:
node_colors[child_name] = child_colors[(i + 8) % len(child_colors)] # You can customize the logic here to assign colors
colors = [node_colors[node] for node in G.nodes()]
# Set figure size
plt.figure(figsize=(30, 30))
# Draw the graph
pos = nx.spring_layout(G, scale=30, seed=seed)
nx.draw_networkx_nodes(G, pos, node_size=10000, node_color=colors, edgecolors='black') # Boundary color set here
nx.draw_networkx_edges(G, pos)
nx.draw_networkx_labels(G, pos, font_size=20)
plt.show()
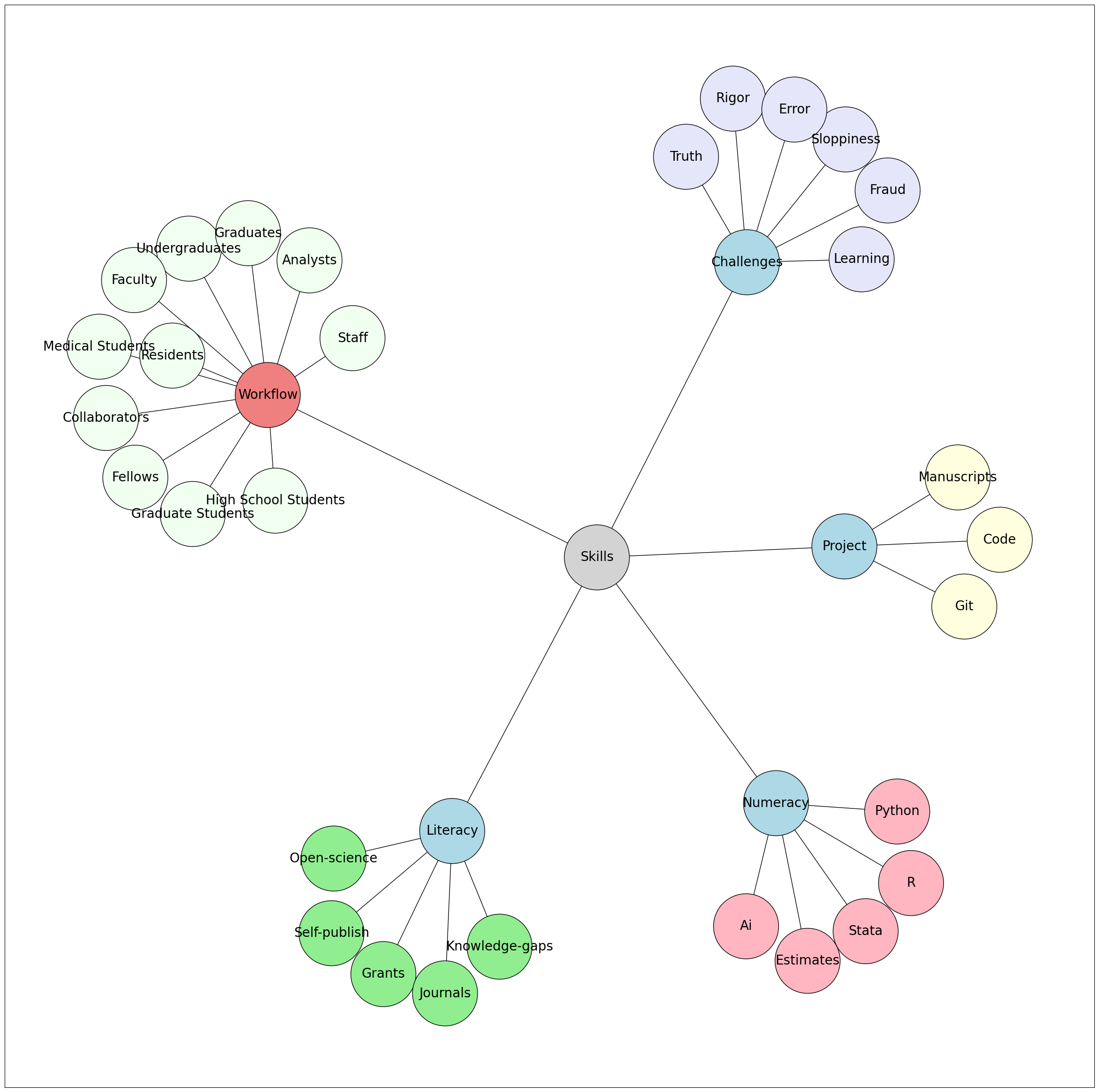
Open Science: A New Paradigm
The Fena platform stands at the forefront of promoting open science, allowing researchers to share their findings, protocols, and knowledge without barriers. By transforming research content into accessible digital formats, Fena makes scientific knowledge transparent, navigable, and impactful. This level of openness amplifies the reach of research and fosters a global community of researchers.
Git Security: Safeguarding Research
Understanding the sensitivity of medical research data, Fena employs rigorous Git security practices that are a specific instance of Public key infrastructure:
Repositories: Distinct
Private
andPublic
repositories ensure data segregation. While public repositories promote open science, private repositories safeguard sensitive or preliminary data.Authentication: Leveraging SSH key authentication, Fena ensures that every user interaction with the platform is secure and traceable, preventing unauthorized data alterations and access.
Encryption: Leveraging the
Public
andPrivate
key infrastructure, data transferred to and from GitHub remain encrypted, ensuring data integrity and confidentiality.
Inclusivity in Collaboration
Fena extends its collaborative ethos to a wide spectrum of contributors, from faculty and seasoned researchers to high school students. This diverse collaboration enriches the research process while also emphasizing ethical considerations and data privacy.
The Role of Infrastructure
Core to Fena’s operations are platforms like Jupyter Book and GitHub. These tools not only offer robust collaboration features but also integrate stringent security measures, protecting sensitive research findings while facilitating open science.
Impact on Healthcare
With a commitment to open science and stringent security protocols, Fena promises a transformative impact on healthcare. Research outcomes become widely available, empowering healthcare professionals with the latest advancements, while data security ensures the confidentiality of sensitive patient data.
Conclusion
The Fena platform heralds a new era where open science and Git security coexist harmoniously. In line with HIPAA guidelines, Fena ensures that no identifiable patient data is stored directly in the repositories. Instead, the platform encourages storing metadata or data references, thereby ensuring that knowledge dissemination doesn’t compromise data security. Regular audits, advanced encryption techniques, and a culture of continuous learning among contributors further fortify the platform against potential breaches, paving the way for a revolution in medical research that is both open and secure.
Show code cell source
import networkx as nx
import matplotlib.pyplot as plt
# Set seed for layout
seed = 35
# Updated directory structure
structure = {
"Challenges": ["Fraud", "Sloppiness", "Rigor", "Learning", "Truth", "Error"],
"Literacy": ["Grants", "Self-publish", "Knowledge-Gaps", "Open-Science", "Journals"],
"Numeracy": ["Stata", "Estimates", "R", "AI", "Python"],
"Project": ["Manuscripts", "Git", "Code", "Security"],
"Security": ["Cloud Key", "HIPAA", "Pairing", "Secure Shell", "Local Key", "Industry Standard", "GitHub Repos"],
"Skills": ["Literacy", "Project", "Numeracy", "Workflow", "Challenges"],
"Workflow": ["High School Students", "Undergraduates", "Graduate Students", "Medical Students", "Residents", "Fellows", "Faculty", "Analysts", "Staff", "Collaborators", "Graduates"],
}
child_colors = ["lightgreen", "lightpink", "lightyellow", 'white', 'honeydew', 'azure', 'lightcoral', 'lightblue', 'lightcyan', 'peachstuff', 'lightsalmon', 'lavender']
G = nx.Graph()
node_colors = {}
# Assign colors to nodes
for i, (parent, children) in enumerate(structure.items()):
parent_name = parent.replace("_", " ").title()
G.add_node(parent_name)
if parent_name == "Skills":
node_colors[parent_name] = 'lightgray'
else:
node_colors[parent_name] = child_colors[i % len(child_colors)]
for child in children:
child_name = child.replace("_", " ").title()
G.add_edge(parent_name, child_name)
if child_name in ["Literacy", "Numeracy", "Project", "Challenges", "Git Security"]:
node_colors[child_name] = 'lightblue'
else:
node_colors[child_name] = child_colors[(i + 11) % len(child_colors)]
colors = [node_colors[node] for node in G.nodes()]
# Set figure size
plt.figure(figsize=(30, 30))
# Draw the graph
pos = nx.spring_layout(G, scale=30, seed=seed)
nx.draw_networkx_nodes(G, pos, node_size=10000, node_color=colors, edgecolors='black') # Boundary color set here
nx.draw_networkx_edges(G, pos)
nx.draw_networkx_labels(G, pos, font_size=20)
plt.show()
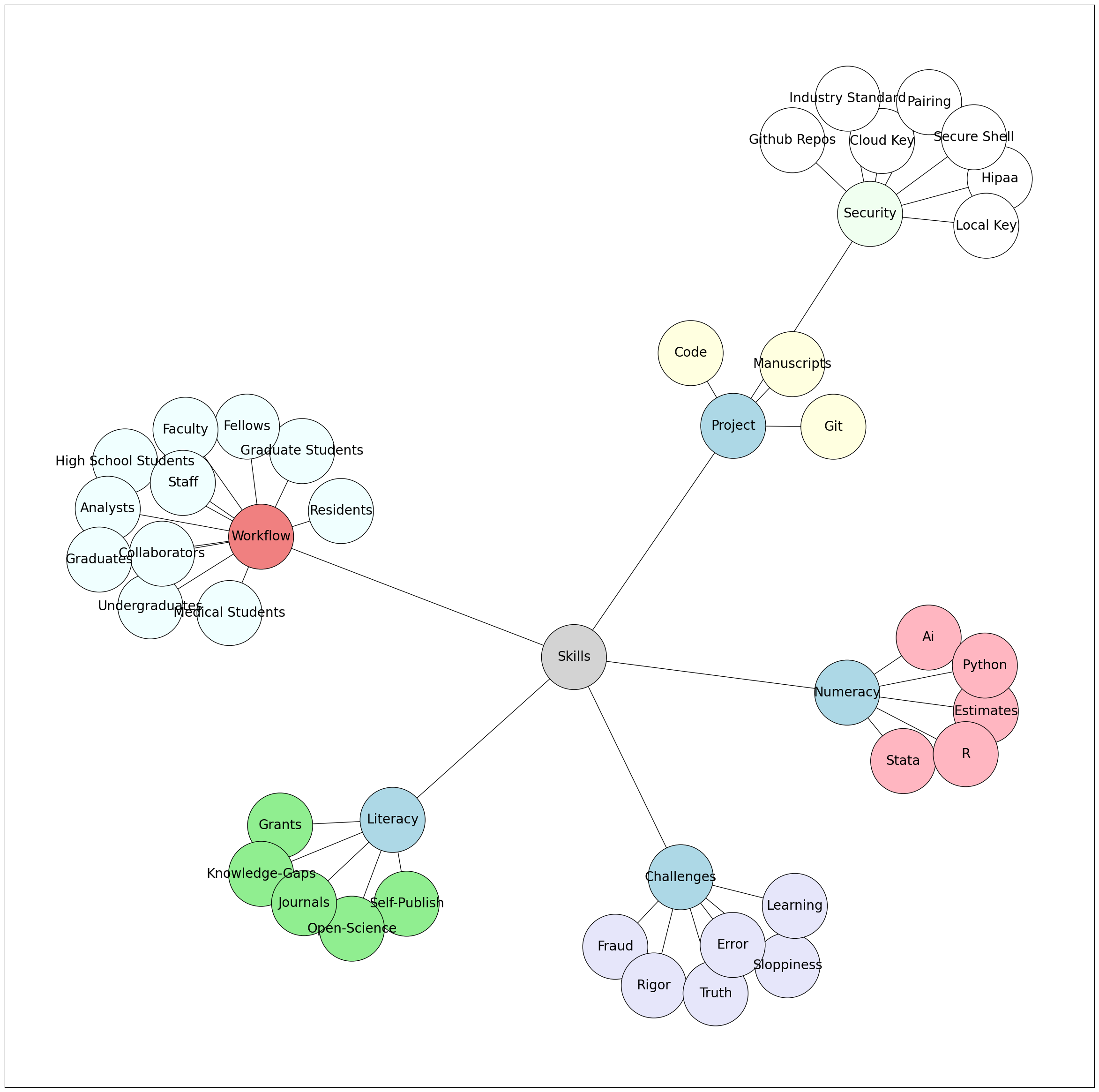
Concerns
A brief breakdown of potential concerns and the provided suggestions:
HIPAA Compliance: HIPAA provides rules to protect the privacy and security of personal health information. Therefore, if any repository (public or private) contains identifiable patient data, it may violate HIPAA regulations.
Suggestion: Ensure no identifiable patient data is stored in the repositories. Instead, data can be stored in a HIPAA-compliant cloud storage, and the platform should allow access to the data only through secure interfaces without allowing the data itself to be downloaded or copied.
Git Repositories: The inherent nature of Git is that every change to the repository is stored in its history. This means that even if sensitive data is deleted in a later commit, its trace might still exist in the repository’s history.
Suggestion: Implement a Git history rewrite or scrubbing tool that allows for the removal of sensitive data from the entire Git history when necessary. Additionally, regularly audit repositories for sensitive data and use pre-commit hooks to scan for and block potential sensitive data from being committed.
Authentication and Encryption: While the platform uses SSH key authentication and public-private key infrastructure, it’s essential to ensure end-to-end encryption, especially if sensitive data is being transmitted.
Suggestion: Regularly review and update the encryption and authentication mechanisms to ensure they adhere to the latest security standards.
Inclusivity in Collaboration: The diverse range of contributors could mean varying levels of awareness about data security and privacy.
Suggestion: Provide mandatory training sessions on data privacy and security to all contributors, ensuring everyone understands the importance of safeguarding sensitive data.
Storage of Data: The main issue revolves around whether data, especially sensitive data, are stored in the repositories.
Suggestion: Instead of storing raw data in repositories, consider storing metadata or data references. If necessary, utilize data tokenization or pseudonymization techniques to protect the actual content of the data.
Epilogue
\( \Large \left\{ \begin{array}{ll} \text{Truth} \\ \text{} \\ \textcolor{gray}{\text{Knowledge}} \ \ \left\{ \begin{array}{l} \textcolor{gray}{\text{Rigor}} \text{} \\ \text{Error} \ \ \ \ \ \ \ \ \ \left\{ \begin{array}{l} \text{Variance} \\ \text{Bias} \end{array} \right. \\ \text{Sloppy} \end{array} \right. \left\{ \begin{array}{l} \text{Explain/Control} \end{array} \right. \\ \text{} \\ \text{Justice} \end{array} \right. \)
Our empirical research journey has covered rigor, error, and sloppiness, as outlined in Chapters 1, 2, and 3. Despite large datasets and rigorous analyses to minimize “unexplained” variance or random errors, biases or systematic errors may persist if we do not actively identify and rectify them. Here we encounter the dilemma between “truth” and “justice,” initially introduced in Chapter 1.
When you reach the “end of the road” in a research domain, it signifies not a stop but the opportunity for new avenues, particularly cross-disciplinary collaborations. Our Fena community aspires to become such a transformative force and advocate for open science in healthcare.
Reflection
We shall not cease from exploration
And the end of all our exploring
Will be to arrive where we started
And know the place for the first time. - T.S. Eliot in Little Gidding